Code in C or C++ to implement the algorithms ALG1, ALG2,and ALG3 (pseudocode algorithms given below). Code shouldgenerate results for each algorithm (to be used as results fortables). Added extra information below on how to implement thealgorithms.
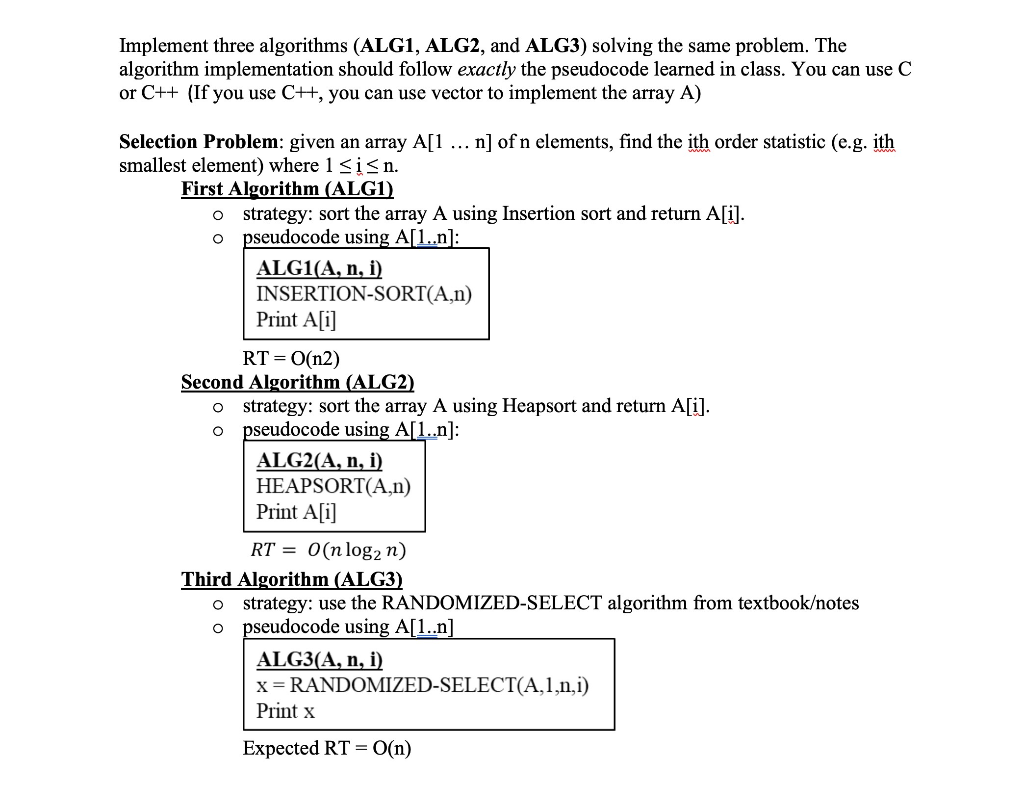
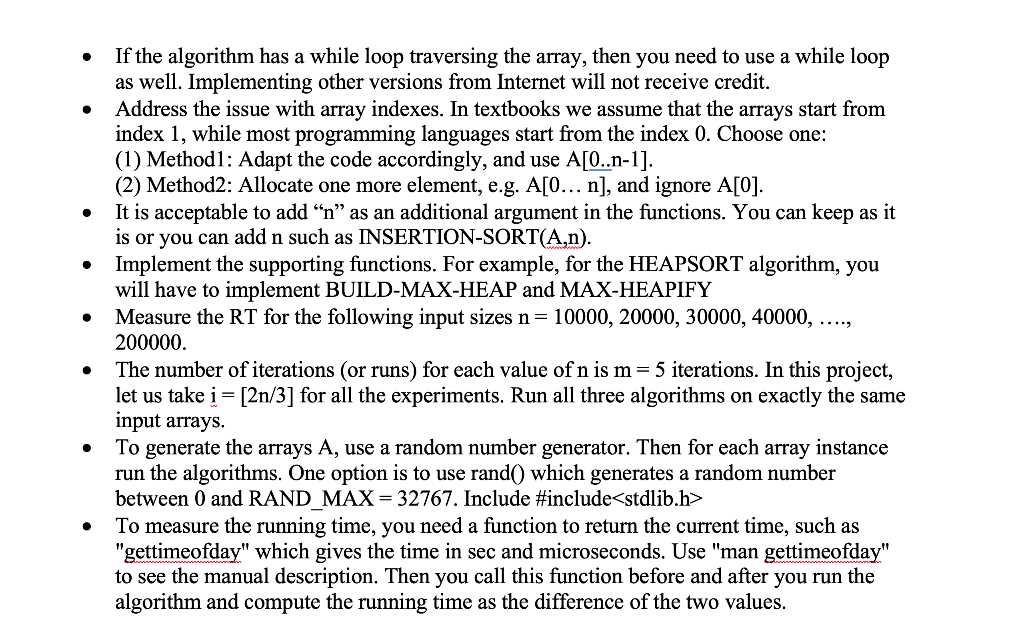
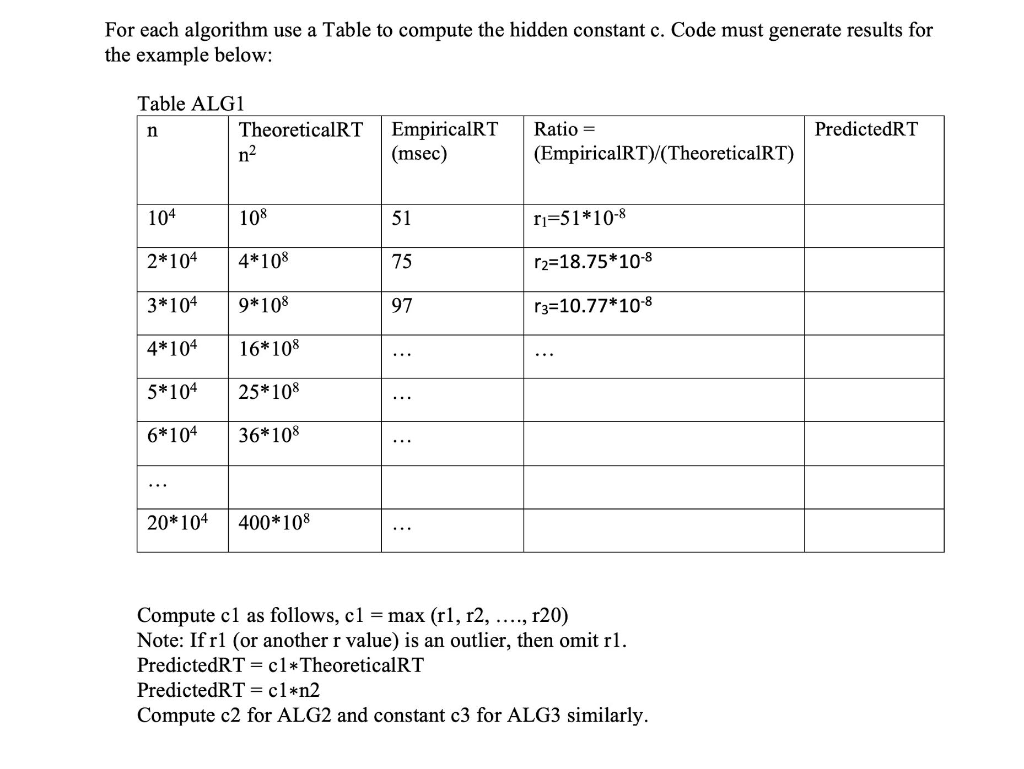
![Insertion Sort (A) for j = 2 to A.length key= A[j] Ninsert A[j] into the sorted sequence A[1.j-1] i=j-1 while i> 0 and A[i] >](https://media.cheggcdn.com/media/227/22757a7e-30d8-4b75-9cf3-b0f6ce7b86e3/phpKMvRk9.png)
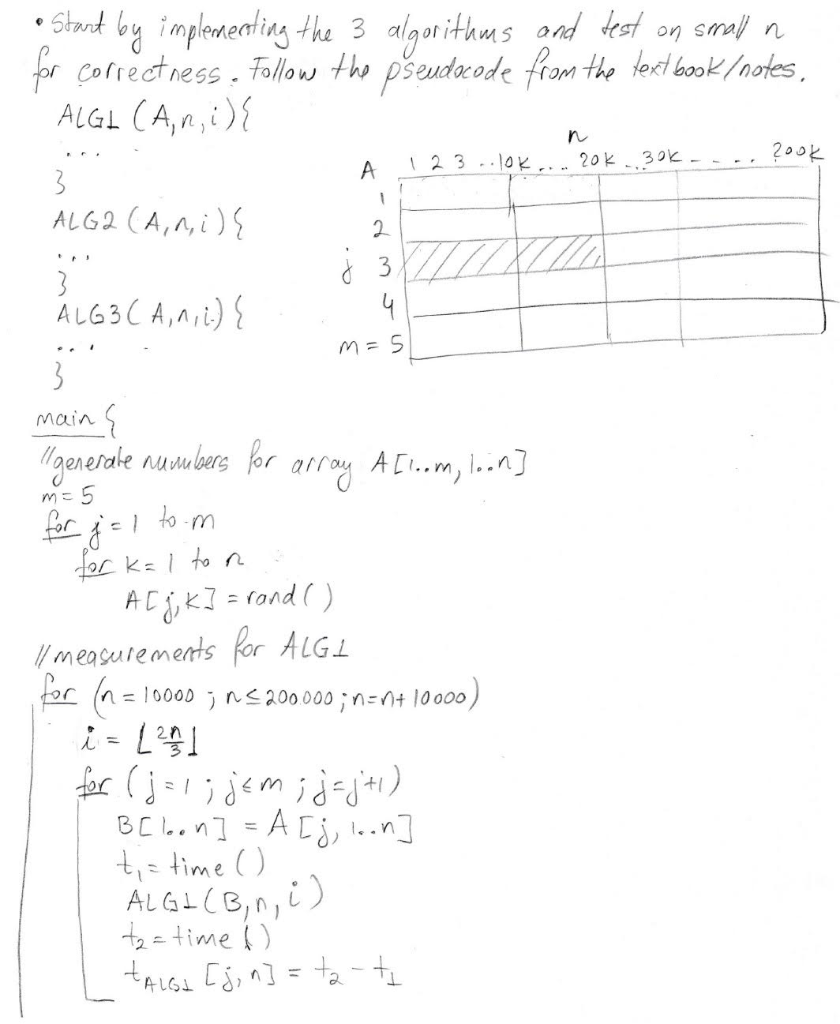
![Il compute the average [n] TALGI EI, n] = LALGA [2, n] tort talgo [m, n] targ-ALGI If this is the value that you plot in the](https://media.cheggcdn.com/media/e49/e49eec61-a41b-494c-809d-9f3b4a2ec127/phpNzJKP4.png)
Implement three algorithms (ALG1, ALG2, and ALG3) solving the same problem. The algorithm implementation should follow exactly the pseudocode learned in class. You can use C or C++ (If you use CH, you can use vector to implement the array A) O Selection Problem: given an array A[1 ... n] of n elements, find the ith order statistic (e.g. ith smallest element) where 1 <i<n. First Algorithm (ALG1) strategy: sort the array A using Insertion sort and return A[i]. pseudocode using A[1..n]: ALG1(A, n, i) INSERTION-SORT(A,n) Print A[i] O RT= O(n2) Second Algorithm (ALG2) strategy: sort the array A using Heapsort and return A[i]. pseudocode using A[1..n]: ALG2(A, n, i) HEAPSORT(A,n) Print A[i] O RT = O(n log2 n) Third Algorithm (ALG3) o strategy: use the RANDOMIZED-SELECT algorithm from textbook/notes o pseudocode using A[1..n] ALG3(A, n, i) x=RANDOMIZED-SELECT(A,1,n,i) Print x Expected RT = O(n) . . If the algorithm has a while loop traversing the array, then you need to use a while loop as well. Implementing other versions from Internet will not receive credit. Address the issue with array indexes. In textbooks we assume that the arrays start from index 1, while most programming languages start from the index 0. Choose one: (1) Methodl: Adapt the code accordingly, and use A[0..n-1). (2) Method2: Allocate one more element, e.g. A[O... n), and ignore A[0]. It is acceptable to add “n” as an additional argument in the functions. You can keep as it is or you can add n such as INSERTION-SORT(A,n). Implement the supporting functions. For example, for the HEAPSORT algorithm, you will have to implement BUILD-MAX-HEAP and MAX-HEAPIFY Measure the RT for the following input sizes n= 10000, 20000, 30000, 40000, ...., 200000. The number of iterations (or runs) for each value of n is m= 5 iterations. In this project, let us take i = [2n/3] for all the experiments. Run all three algorithms on exactly the same input arrays. To generate the arrays A, use a random number generator. Then for each array instance run the algorithms. One option is to use rand() which generates a random number between 0 and RAND_MAX= 32767. Include #include<stdlib.h> To measure the running time, you need a function to return the current time, such as "gettimeofday" which gives the time in sec and microseconds. Use "man gettimeofday" to see the manual description. Then you call this function before and after you run the algorithm and compute the running time as the difference of the two values. For each algorithm use a Table to compute the hidden constant c. Code must generate results for the example below: Table ALG1 n TheoreticalRT n2 PredictedRT EmpiricalRT (msec) Ratio = (EmpiricalRT)/(TheoreticalRT) 104 108 51 ri=51*10-8 2*104 4*108 75 r2=18.75*10-8 3*104 9*108 97 r3=10.77*10-8 4*104 16*108 5*104 25*108 6*104 36*108 20*104 400*108 Compute cl as follows, cl = max (rl, r2, ...., r20) Note: Ifrl (or another r value) is an outlier, then omit rl. PredictedRT = cl* TheoreticalRT PredictedRT = cl*n2 Compute c2 for ALG2 and constant c3 for ALG3 similarly. Insertion Sort (A) for j = 2 to A.length key= A[j] Ninsert A[j] into the sorted sequence A[1.j-1] i=j-1 while i> 0 and A[i] > key A[i+1] = A[i] i=i-1 A[i+1] = key Heapsort (A) n= A.heap-size = A.length BUILD-MAX-HEAP(A) for i= n down to 2 exchange A[1] with A[i] A.heap-size = A.heap-size-1 MAX-HEAPIFY (A,1) RT Analysis RT = O(nlgn) BUILD-MAX-HEAP (A) n=A.heap-size = A.length for i=La| down to 1 MAX-HEAPIFY (A,i) MAX-HEAPIFY (A,i) n= A.heap-size (=LEFT(i) r=RIGHT(i) if ( sn and A[q> A[i] largest =( else largest = i ifr Sn and A[r] > A[largest] largest=r if largest #i exchange A[i] with A[largest] MAX-HEAPIFY (A, largest) Simple bound: RT = O(nlgn) as there are O(n) calls to MAX-HEAPIFY which takes O(Ign) RANDOMIZED-SELECT (A, p, r, i) if p ==r return A[p] q=RANDOMIZED-PARTITION (A, p, r) k=q-p+1 if i==k return A[q] else if i <k return RANDOMIZED-SELECT (A, p, q-1, i) else //i>k return RANDOMIZED-SELECT (A, 2+1, r, i-k) RT = O(h) where h is the height of the node i Since h= O(Ign), RT is also expressed as O(Ign) initial call: RANDOMIZED-SELECT (A, 1, A.length, i) RANDOMIZED-PARTITION (A, p, r) i=RANDOM (p, r) exchange A[r] with A[i] return PARTITION (A, p, r) Worst-case: T(n) = O(na) Best-case: T(n) = O(n) • Start by implementing the 3 algorithms and test on small in for correctness - Fallow the pseudocode from the text book /notes, ALGI (A, n, i) { n 20k 123.OK 30k 2ook A 3 ALGQ (A, n, i) { 2 2 3 4 ÁLG3 (A, n, i) { m = 5 3 main { m=5 for j = 1 m for (n= (generale numbers for array A[loom, loon to for kalton AC{k} = rond Il measurements for ALG2 1= 10000 in 5 200.000;n=n+ 10000) 100) i = £241 for (j=1; jem ; j =j+1) BC.nl - And t = time () ALG!CB, n, i) to a time & tales [j, n] = ta-ti Il compute the average [n] TALGI EI, n] = LALGA [2, n] tort talgo [m, n] targ-ALGI If this is the value that you plot in the graph "Empirical RT /repeat for ALGO Il repeat for AL63 Note: when array A, feel free to use dynamic alla cateon using pointers for C, vector' Por'ctt, etc. you allocate the array